LDR
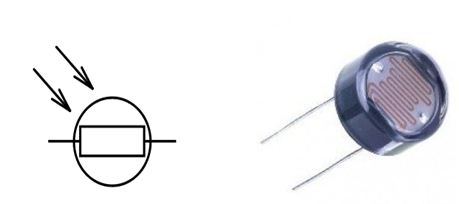

Types of Light Dependent Resistors

Applications
/* Name : main.c * Purpose : Source code for LDR Interfacing with AT89C52. * Author : Gemicates * Date : 2017-06-15 * Website : www.gemicates.org * Revision : None */ //Program to check the working of LDR using LCD on its output port. #include <REGX52.H> #define lcd P0 // LCD data pins sbit rs = P1^5; // register select pin sbit rw= P1^6; // read write pin sbit e = P1^7; // enable pin #define input P2 // Input port to read the values of ADC #define output P0 // Output port, connected to LED's. sbit wr= P1^1; // Write pin. It is used to start the conversion. sbit rd= P1^0; // Read pin. It is used to extract the data from internal register to the output pins of ADC. sbit intr= P1^2; // Interrupt pin. This is used to indicate the end of conversion. It goes low when conversion is complete. unsigned char number,value,v1,v2,v3; void delay(unsigned int msec ) // The delay function provides delay in msec. { int i,j ; for(i=0;i<msec;i++) for(j=0;j<1275; j++); } void lcddata(char t) // lcd data function { rs=1; lcd=t; rw=0; e=1; delay(1); e=0; } void cmd(unsigned char c) // lcd command function { lcd=c; rs=0; rw=0; e=1; delay(1); e=0; } void com() // lcd initialization function { cmd(0x38); delay(10); cmd(0x0c); delay(10); cmd(0x01); delay(10); } void string(char *d) // lcd string print function { while(*d !=0) { lcddata(*d++); } } void BCD() // BCD conversion function { number=output; value=number; v1=value/100+0x30; v2=value%100/10+0x30; v3=value%10+0x30; string("value :"); cmd(0x88); lcddata(v1); lcddata(v2); lcddata(v3); // value=number*19.62; // v1=value/100+0x30; // v2=value%100/10+0x30; // v3=value%10+0x30; // cmd(0xc0); // // string("volts :"); // cmd(0xc8); // lcddata(v1); // lcddata('.'); // lcddata(v2); // lcddata(v3); } void adc() // Function to read the values from ADC0804 and display on the LCD. { rd=1; wr=0; delay(1); wr=1; while(intr==1); rd=0; output=input; BCD(); delay(1); intr=1; } void main() { input=0xff; // Declare port 2 as input port. while(1) { com(); adc(); delay(100); } }